2020. 3. 2. 19:59ㆍ카테고리 없음
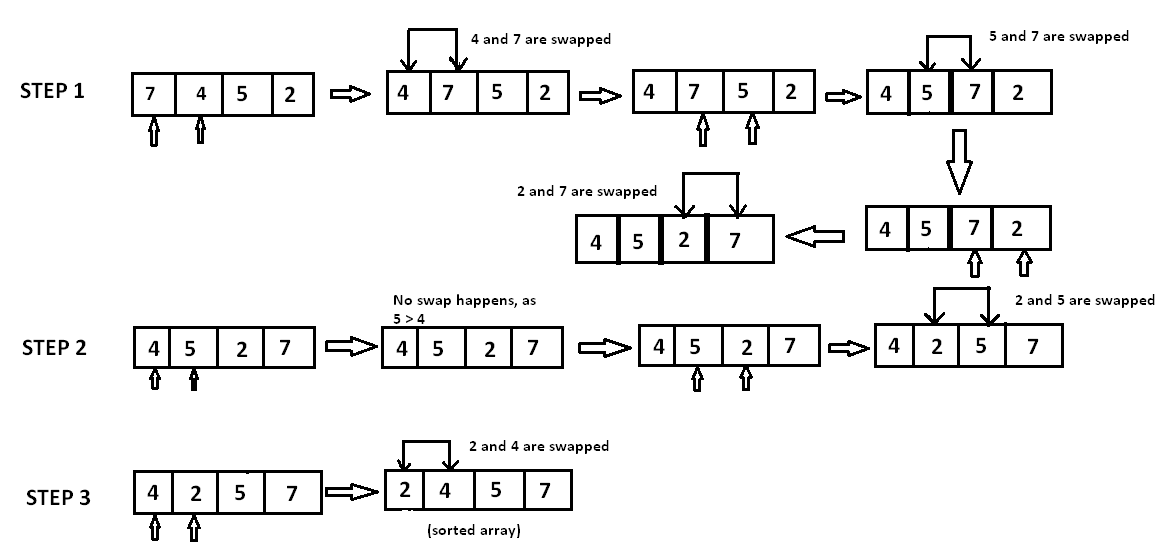
Program Greetings;constmessage = ' Welcome to the world of Pascal ';typename = string;varfirstname, surname: name;beginwriteln('Please enter your first name: ');readln(firstname);writeln('Please enter your surname: ');readln(surname);writeln;writeln(message, ' ', firstname, ' ', surname);end.When the above code is compiled and executed, it produces the following result −Please enter your first name:JohnPlease enter your surname:SmithWelcome to the world of Pascal John SmithEnumerated VariablesYou have seen how to use simple variable types like integer, real and boolean. Now, let's see variables of enumerated type, which can be defined as −varvar1, var2.: enum-identifier;When you have declared an enumerated type, you can declare variables of that type. For example,typemonths = (January, February, March, April, May, June, July, August, September, October, November, December);Varm: months.M:= January;The following example illustrates the concept −. Program exEnumeration;typebeverage = (coffee, tea, milk, water, coke, limejuice);vardrink:beverage;beginwriteln('Which drink do you want?' );drink:= limejuice;writeln('You can drink ', drink);end.When the above code is compiled and executed, it produces the following result −Which drink do you want?You can drink limejuiceSubrange VariablesSubrange variables are declared as −varsubrange-name: lowerlim.
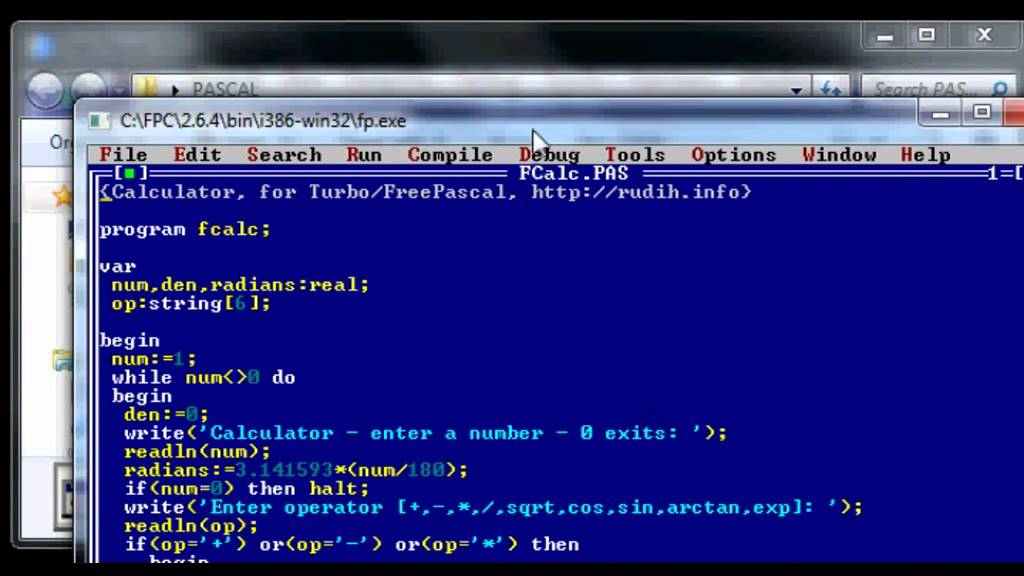
Uperlim;Examples of subrange variables are −varmarks: 1. 100;grade: 'A'. 25;The following program illustrates the concept −. Program recursiveFibonacci;vari: integer;function fibonacci(n: integer): integer;beginif n=1 thenfibonacci:= 0else if n=2 thenfibonacci:= 1elsefibonacci:= fibonacci(n-1) + fibonacci(n-2);end;beginfor i:= 1 to 10 dowrite(fibonacci (i), ' ');end.When the above code is compiled and executed, it produces the following result −0 1 1 23 5 8 13 21 34Arguments of a SubprogramIf a subprogram ( function or procedure) is to use arguments, it must declare variables that accept the values of the arguments. These variables are called the formal parameters of the subprogram.The formal parameters behave like other local variables inside the subprogram and are created upon entry into the subprogram and destroyed upon exit.While calling a subprogram, there are two ways that arguments can be passed to the subprogram − Sr.NoCall Type & Description1This method copies the actual value of an argument into the formal parameter of the subprogram. In this case, changes made to the parameter inside the subprogram have no effect on the argument.2This method copies the address of an argument into the formal parameter.
Inside the subprogram, the address is used to access the actual argument used in the call. This means that changes made to the parameter affect the argument.By default, Pascal uses call by value to pass arguments. In general, this means that code within a subprogram cannot alter the arguments used to call the subprogram. The example program we used in the chapter 'Pascal - Functions' called the function named max using call by value.Whereas, the example program provided here ( exProcedure) calls the procedure findMin using call by reference. Pascal - Variable ScopeA scope in any programming is a region of the program where a defined variable can have its existence and beyond that variable cannot be accessed.
There are three places, where variables can be declared in Pascal programming language −.Inside a subprogram or a block which is called local variables.Outside of all subprograms which is called global variables.In the definition of subprogram parameters which is called formal parametersLet us explain what are local and global variables and formal parameters. Local VariablesVariables that are declared inside a subprogram or block are called local variables.
They can be used only by statements that are inside that subprogram or block of code. Local variables are not known to subprograms outside their own. Following is the example using local variables. Here, all the variables a, b and c are local to program named exLocal. Program exString;uses sysutils;varstr1, str2, str3: ansistring;str4: string;len: integer;beginstr1:= 'Hello ';str2:= 'There!'
;(. copy str1 into str3.)str3:= str1;writeln('appendstr( str3, str1): ', str3 );(. concatenates str1 and str2.)appendstr( str1, str2);writeln( 'appendstr( str1, str2) ', str1 );str4:= str1 + str2;writeln('Now str4 is: ', str4);(. total lenghth of str4 after concatenation.)len:= byte(str40);writeln('Length of the final string str4: ', len);end.When the above code is compiled and executed, it produces the following result −appendstr( str3, str1): Helloappendstr( str1, str2): Hello There!Now str4 is: Hello There! There!Length of the final string str4: 18Pascal String Functions and ProceduresPascal supports a wide range of functions and procedures that manipulate strings. These subprograms vary implement-wise. Program exBoolean;varexit: boolean;choice: char;beginwriteln('Do you want to continue?
');writeln('Enter Y/y for yes, and N/n for no');readln(choice);if(choice = 'n') thenexit:= trueelseexit:= false;if (exit) thenwriteln(' Good Bye!' )elsewriteln('Please Continue');readln;end.When the above code is compiled and executed, it produces the following result −Do you want to continue?Enter Y/y for yes, and N/n for noNGood Bye!YPlease ContinuePascal - ArraysPascal programming language provides a data structure called the array, which can store a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.Instead of declaring individual variables, such as number1, number2., and number100, you declare one array variable such as numbers and use numbers1, numbers2, and., numbers100 to represent individual variables. A specific element in an array is accessed by an index.All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.Please note that if you want a C style array starting from index 0, you just need to start the index from 0, instead of 1. Program exPointers;varnumber: integer;iptr: ^integer;beginnumber:= 100;writeln('Number is: ', number);iptr:= @number;writeln('iptr points to a value: ', iptr^);iptr^:= 200;writeln('Number is: ', number);writeln('iptr points to a value: ', iptr^);end.When the above code is compiled and executed, it produces the following result −Number is: 100iptr points to a value: 100Number is: 200iptr points to a value: 200Printing a Memory Address in PascalIn Pascal, we can assign the address of a variable to a pointer variable using the address operator (@). We use this pointer to manipulate and access the data item.
However, if for some reason, we need to work with the memory address itself, we need to store it in a word type variable.Let us extend the above example to print the memory address stored in the pointer iptr −. Program exPointers;varnumber: integer;iptr: ^integer;y: ^word;beginnumber:= 100;writeln('Number is: ', number);iptr:= @number;writeln('iptr points to a value: ', iptr^);iptr^:= 200;writeln('Number is: ', number);writeln('iptr points to a value: ', iptr^);y:= addr(iptr);writeln(y^);end.When the above code is compiled and executed, it produces the following result −Number is: 100iptr points to a value: 100Number is: 200iptr points to a value: 20045504NIL PointersIt is always a good practice to assign a NIL value to a pointer variable in case you do not have exact address to be assigned. This is done at the time of variable declaration. A pointer that is assigned NIL points to nowhere. Consider the following program −. Program exPointers;varnumber: integer;iptr: ^integer;y: ^word;beginiptr:= nil;y:= addr(iptr);writeln('the vaule of iptr is ', y^);end.When the above code is compiled and executed, it produces the following result −The value of ptr is 0To check for a nil pointer you can use an if statement as follows −if(ptr nill )then (. succeeds if p is not null.)if(ptr = nill)then (.

succeeds if p is null.)Pascal Pointers in DetailPointers have many but easy concepts and they are very important to Pascal programming. Program exMemory;varname: array1.100 of char;description: ^string;desp: string;beginname:= 'Zara Ali';desp:= 'Zara ali a DPS student.' ;description:= getmem(30);if not assigned(description) thenwriteln('Error - unable to allocate required memory')elsedescription^:= desp;(. Suppose you want to store bigger description.)description:= reallocmem(description, 100);desp:= desp + ' She is in class 10th.' ;description^:= desp;writeln('Name = ', name );writeln('Description: ', description^ );freemem(description);end.When the above code is compiled and executed, it produces the following result −Name = Zara AliDescription: Zara ali a DPS student.
Selection Sort Pascal
She is in class 10thMemory Management FunctionsPascal provides a hoard of memory management functions that is used in implementing various data structures and implementing low-level programming in Pascal. Many of these functions are implementation dependent.
Program TimeDemo;uses sysutils;beginwriteln ('Current time: ',TimeToStr(Time));end.When the above code was compiled and executed, it produces the following result −Current time: 18:33:08The Date function returns the current date in TDateTime format. The TDateTime is a double value, which needs some decoding and formatting. The following program demonstrates how to use it in your program to display the current date −Program DateDemo;uses sysutils;varYY,MM,DD: Word;beginwriteln ('Date: ',Date);DeCodeDate (Date,YY,MM,DD);writeln (format ('Today is (DD/MM/YY):%d/%d/%d ',dd,mm,yy));end.When the above code was compiled and executed, it produces the following result −Date: 4.00000E+004Today is (DD/MM/YY):23/7/2012The Now function returns the current date and time −.
FilternoneOutput: 11 11 2 11 3 3 11 4 6 4 1This method can be optimized to use O(n) extra space as we need values only from previous row. So we can create an auxiliary array of size n and overwrite values. Following is another method uses only O(1) extra space.Method 3 ( O(n^2) time and O(1) extra space )This method is based on method 1.
We know that ith entry in a line number line is Binomial Coefficient C(line, i) and all lines start with value 1. The idea is to calculate C(line, i) using C(line, i-1).
It can be calculated in O(1) time using the following.C(line, i) = line! / ( (line-i)! )C(line, i-1) = line! / ( (line - i + 1)! )We can derive following expression from above two expressions.C(line, i) = C(line, i-1). (line - i + 1) / iSo C(line, i) can be calculated from C(line, i-1) in O(1) time.